23854번: The Battle of Giants
The famous programming contest organizer decided to hold competition for champions "The Battle of Giants". There are two teams competing in the battle. Several matches are organized for the competition. Each match can end with a win for one of the teams, o
www.acmicpc.net
문제 설명
The famous programming contest organizer decided to hold competition for champions "The Battle of Giants". There are two teams competing in the battle. Several matches are organized for the competition.
Each match can end with a win for one of the teams, or with a draw. When a team wins the match it scores 3 points, in this case the opposite team doesn't score any point. In the case of a draw, teams score 1 point each. After all the matches end, the final score aaa:bbb is calculated: aaa and bbb --- the number of points scored by the first and second team, respectively.
For example, if the first match is won by the first team, the second match ended with a draw, and the third match is also won by the first team, the final score is 7:1.
You are given the final score. Find out whether the given score is possible, and what is the minimum number of matches could be. Print the number of matches won by the first team, the number of matches ended with a draw, and the number of matches won by the second team.
입력 )
The first line contains a single integer a --- the number of points scored by the first team.
The first line contains a single integer b --- the number of points scored by the second team.
The given aa and bb are nonnegative and don't exceed $10^9$.
출력 )
If the given final score isn't possible, print -1.
Otherwise, print three integers: the number of matches won by the first team, the number of matches ended with a draw, and the number of matches won by the second team, respectively. Find any solution with the minimum number of matches played.
예제 )
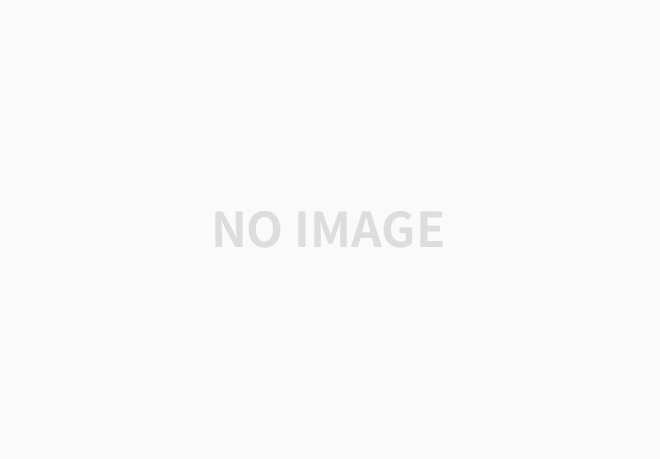
❕ 문제 풀이
A와 B팀이 배틀을 하는데, 이기면 이긴팀만 3점을 득점하고, 비기면 양쪽팀에 1점이 부여된다.
A & B팀의 최종 점수를 보고 A팀이 이긴 횟수, 무승부 횟수, B팀이 이긴 횟수를 출력한다.
- a팀이 이긴 횟수, 무승부 횟수, b팀이 이깃 횟수를 모두 0으로 선언해둔다.
- 팀의 점수가 3점 미만인 2점, 1점, 0점이라면, 단 한 번도 이기지 못했다는 것을 알 수 있다.
- 팀의 점수가 3점 이상이라면, 3으로 나눈 몫만큼 이겼다고 생각할 수 있다.
- 각 팀의 이긴 횟수를 구해주고 남은 나머지가 0점 이상이고 두 팀의 점수가 동일하다면, 무승부를 통해 얻은 점수임을 알 수 있다.
1.
int a = Integer.parseInt(br.readLine());
int b = Integer.parseInt(br.readLine());
int a_win = 0;
int draw = 0;
int b_win = 0;
공백없이 두 팀의 점수를 각각 입력받고, 후에 계산해줄 횟수들을 0으로 초기화해준다.
2.
if (a >= 3) {
a_win = a / 3;
a = a % 3;
}
if (b >= 3) {
b_win = b / 3;
b = b % 3;
}
각 팀의 점수가 3점 이상 득점했다면, 이긴 횟수가 분명 있다고 판단하여, 3으로 나누어 몫을 통해 횟수를 구해준다. 그 후, 남은 점수를 위해 나머지를 구한다.
3.
if (a == b) {
draw = a;
bw.write(a_win +" "+ draw +" "+ b_win);
} else {
bw.write(String.valueOf(-1)); // bw에서 정수형 출력 시, String을로 감싸기
}
각 팀의 남은 점수가 둘의 점수가 동일하다면, 무승부를 통해 얻은 점수라고 판단할 수 있다. 무승부를 통해 얻을 수 있는 점수는 1점이므로, a팀의 남은 점수를 더해주어 횟수를 구해준다.
둘의 점수가 동일하지 않다면, 불가능한 입력값이므로 -1을 출력해준다.
✅ [ CODE ]
import java.io.*;
public class Main{
public static void main(String[] args) throws IOException {
BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
BufferedWriter bw = new BufferedWriter(new OutputStreamWriter(System.out));
int a = Integer.parseInt(br.readLine());
int b = Integer.parseInt(br.readLine());
int a_win = 0;
int draw = 0;
int b_win = 0;
if (a >= 3) {
a_win = a / 3;
a = a % 3;
}
if (b >= 3) {
b_win = b / 3;
b = b % 3;
}
if (a == b) {
draw = a;
bw.write(a_win +" "+ draw +" "+ b_win);
} else {
bw.write(String.valueOf(-1)); // bw에서 정수형 출력 시, String을로 감싸기
}
bw.flush();
br.close();
bw.close();
}
}