[Java] 반복문, 배열, 예외 처리 || Java Programming
1. 반복문
- for문
초기문은 시작할 때 한 번만 수행된다. 콤마로 구분되며, 세미콜론이 있어야 한다.
조건식에 true가 있거나 비어 있으면 무한 반복이 된다.
반복 후 작업 문에는 여러 문장을 나열할 수 있다. (아래에 예시)
for (i = 0; i < 10; i++, System.out.println(i)) {
...
}
- while문
조건식이 없으면, 컴파일 오류가 발생한다.
조건식에 사용되는 변수는 while문 실행 전에 초기화되어 있어야 한다.
while (true) {
if(조건식){
break;
}
}
- do while문
작업문 실행 후 조건식을 검사하므로 작업문이 한 번은 반드시 실행된다.
do {
...
} while (조건식)
- for vs. while
반복의 횟수, 범위가 명확한 경우 for문을 이용한다.
반복 횟수를 알 수 없고, 진행되면서 결정되는 경우엔 while문과 do while문을 이용한다.
무한 반복에 빠지지 않도록 조건식에 주의해야 한다.
- continue vs. break
continue문은 반복문을 빠져나가지 않고, 다음 반복으로 넘어갈 수 있도록 한다.

break문은 하나의 반복문을 즉시 빠져나간다.

- for - each문
advanced for이라고도 불린다. 배열과 같은 iterate 객체에서 각 원소를 순차적으로 접근하는 것이다.
int n [] = {1, 2, 3};
int sum = 0;
for (int i : n){
sum += i;
}
System.out.println(sum);
2. 배열
배열이란, 인덱스와 그에 대응하는 데이터로 이루어진 연속적인 자료 구조로, 같은 종류의 데이터들이 순차적으로 저장된다.
- 선언 및 초기화
(1) 배열에 대한 레퍼런스 변수 선언
int array [];
int [] array2;
array는 배열 공간에 대한 주소를 가지고 있으며, 공간 할당을 해주지 않았으므로 null이다.
배열의 주소를 레퍼런스라고 부르며, 배열에 대한 주소를 가지는 변수는 레퍼런스 변수이다.
array는 레퍼런스 변수이다.
배열을 선언해줄 때, 배열의 크기를 지정해주면 컴파일 오류가 발생한다.
int array[5]; // 컴파일 오류
(2) 배열의 저장 공간 할당
array = new int [5];
new 연산자를 이용하여 배열을 생성하며 크기를 지정한다.
정수 5개의 공간을 할당받고 배열에 대한 주소를 array에 저장한다.
이제, array를 배열로 사용할 수 있다.
선언과 생성을 동시에 할 수 있고, 다음과 같이 초기화된 배열을 만들 수 있다.
int array[] = new int [5];
int array2[] = { 1, 2, 3, 4, 5 };
- 레퍼런스 치환 & 배열 공유
레퍼런스 변수와 배열 공간이 분리되어 있다.
int array[] = new int[5];
int array2[] = array; // 레퍼런스 치환
이 치환으로 array 배열이 복사되는 것이 아니라, 배열에 대한 주소만 복사된다.
array와 array2는 동일한 배열을 공유하게 된다.

3. 예외 처리
모든 예외 클래스는 java.lang.Exception 클래스를 상속받는다.
- 자주 발생하는 예외
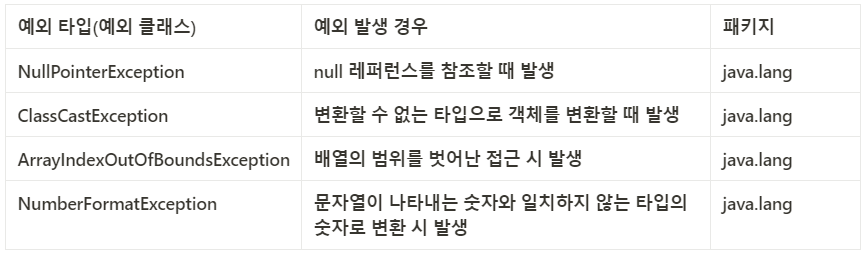
- try ~ catch ~ finally문
try {
예외가 발생할 가능성이 있는 실행문
}
catch ( 예외 타입 ) {
예외 처리문
}
finally {
예외 발생과 상관없이 무조건 실행되는 문장
}
실습 문제
import java.util.InputMismatchException;
import java.util.Scanner;
public class Array_Repeat_Exception_Examples {
/*
배열, 반복문, 예외 처리 연습
*/
Scanner sc = new Scanner(System.in);
void Ex01_3() {
System.out.println("---------[Ex01_3]---------");
int sum = 0;
for (int i = 0; i < 100; i += 2) {
sum += i;
}
System.out.println(sum);
}
void Ex01_4() {
System.out.println("---------[Ex01_4]---------");
int sum = 0, i = 0;
do {
sum += i;
i += 2;
} while (i < 100);
System.out.println(sum);
}
void Ex02() {
System.out.println("---------[Ex02]---------");
int n[][] = {{1}, {1, 2, 3}, {1}, {1, 2, 3, 4}, {1, 2}};
for (int i = 0; i < n.length; i++) {
for (int j = 0; j < n[i].length; j++) {
System.out.print(n[i][j] + " ");
}
System.out.println();
}
}
void Ex03() {
System.out.println("---------[Ex03]---------");
System.out.print("정수를 입력하시오>>");
int cnt = sc.nextInt();
for (int i = cnt; i > 0; i--) {
for (int j = 0; j < i; j++) {
System.out.print("*");
}
System.out.println();
}
}
void Ex04() {
System.out.println("---------[Ex04]---------");
System.out.print("소문자 알파벳 하나를 입력하시오>>");
/*
scanner를 통해 입력받는 것은 String 타입이다.
charAt() : 문자열 중 하나를 골라 char 타입으로 변환. (인덱스로 0을 넣어주어, 첫 글자 선택)
*/
char alpha = sc.next().charAt(0);
for (int i = 0; i <= alpha - 'a'; i++) {
for (char j = 'a'; j <= alpha - i; j++) {
System.out.print(j);
}
System.out.println();
}
}
void Ex05() {
System.out.println("---------[Ex05]---------");
System.out.print("양의 정수 10개를 입력하시오>>");
int[] arr = new int[10];
for (int i = 0; i < 10; i++) {
arr[i] = sc.nextInt();
}
System.out.print("3의 배수는 ");
for (int i : arr) {
if (i % 3 == 0) {
System.out.print(i + " ");
}
}
}
void Ex06() {
System.out.println("---------[Ex06]---------");
System.out.print("금액을 입력하시오>>");
int money = sc.nextInt();
int[] unit = {50000, 10000, 1000, 500, 100, 50, 10, 1};
for (int i : unit) {
if (money / i != 0) {
System.out.println(i + "원 짜리 : " + money / i + "개");
money %= i;
}
}
}
void Ex07() {
System.out.println("---------[Ex07]---------");
int sum = 0;
System.out.print("랜덤한 정수들 : ");
for (int i = 0; i < 10; i++) {
int x = (int) (Math.random() * 10 + 1); // 1부터 10까지의 범위의 정수 랜덤 생성
System.out.print(x + " ");
sum += x;
}
System.out.println();
System.out.print("평균은 " + (double) sum / 10);
}
void Ex08() {
System.out.println("---------[Ex08]---------");
System.out.print("정수 몇개?");
int x = sc.nextInt();
int[] arr = new int[x];
for (int i = 0; i < x; i++) {
int value = (int) (Math.random() * 100 + 1);
for (int j = 0; j < i; j++) {
if (arr[i] == value) {
i--;
break;
}
}
arr[i] = value;
}
for (int i = 0; i < x; i++) {
if (i != 0 && i % 10 == 0) {
System.out.println();
}
System.out.print(arr[i] + " ");
}
}
void Ex09() {
System.out.println("---------[Ex09]---------");
int[][] arr = new int[4][4];
for (int i = 0; i < arr.length; i++) {
for (int j = 0; j < arr[1].length; j++) {
arr[i][j] = (int) (Math.random() * 10 + 1);
System.out.print(arr[i][j] + "\t");
}
System.out.println();
}
}
void Ex10() {
System.out.println("---------[Ex10]---------");
int[][] arr = new int[4][4];
for (int i = 0; i < arr.length; i++) {
for (int j = 0; j < arr[1].length; j++) {
arr[i][j] = (int) (Math.random() * 10 + 1);
}
}
for (int i = 0; i < 6; i++) {
int x = (int) (Math.random() * 4);
int y = (int) (Math.random() * 4);
if (arr[x][y] != 0) {
arr[x][y] = 0;
} else {
i--;
}
}
for (int i = 0; i < arr.length; i++) {
for (int j = 0; j < arr[1].length; j++) {
System.out.print(arr[i][j] + "\t");
}
System.out.println();
}
}
void Ex13() {
System.out.println("---------[Ex13]---------");
for (int i = 0; i < 38; i++) {
int x = i / 10;
int y = i % 10;
if (x == 3 || x == 6 || x == 9) {
if (y == 3 || y == 6 || y == 9) {
System.out.println(i + "박수 짝짝");
} else {
System.out.println(i + "박수 짝");
}
}
}
}
void Ex14() {
System.out.println("---------[Ex14]---------");
String course[] = {"Java", "C++", "HTML5", "컴퓨터구조", "안드로이드"};
int score[] = {95, 88, 76, 62, 55};
while (true) {
System.out.print("과목 이름>>");
String command = sc.next();
if (command.equals("그만")) {
break;
}
int cnt = 0;
for (int i = 0; i < course.length; i++) {
if (command.equals(course[i])) {
System.out.println(command + "의 점수는 " + score[i]);
cnt += 1;
break;
}
}
if (cnt == 0) {
System.out.println("없는 과목입니다.");
}
}
}
void Ex15() {
System.out.println("---------[Ex15]---------");
while(true) {
System.out.print("곱하고자 하는 두 수 입력>>");
try {
int n = sc.nextInt();
int m = sc.nextInt();
System.out.print(n + "X" + m +"="+ n*m);
break;
}catch(InputMismatchException e) {
System.out.println("실수는 입력하면 안됩니다.");
sc.nextLine();
continue;
}
}
}
void Ex16() {
System.out.println("---------[Ex16]---------");
System.out.println("컴퓨터와 가위 바위 보 게임을 합니다.");
String str[] = {"가위", "바위", "보"};
while (true) {
System.out.print("가위 바위 보!>>");
String command = sc.next();
if (command.equals("그만")) {
break;
}
int computer = (int) (Math.random() * 3);
System.out.print("사용자 = " + command + ", 컴퓨터 = " + str[computer] + ", ");
if (computer == 0) {
if (command.equals("가위")) {
System.out.println("비겼습니다.");
} else if (command.equals("바위")) {
System.out.println("사용자가 이겼습니다.");
} else if (command.equals("보")) {
System.out.println("컴퓨터가 이겼습니다.");
} else {
System.out.println("옳은 명령어를 입력해주세요.");
}
} else if (computer == 1) {
if (command.equals("가위")) {
System.out.println("컴퓨터가 이겼습니다.");
} else if (command.equals("바위")) {
System.out.println("비겼습니다.");
} else if (command.equals("보")) {
System.out.println("사용자가 이겼습니다.");
} else {
System.out.println("옳은 명령어를 입력해주세요.");
}
} else {
if (command.equals("가위")) {
System.out.println("사용자가 이겼습니다.");
} else if (command.equals("바위")) {
System.out.println("컴퓨터가 이겼습니다.");
} else if (command.equals("보")) {
System.out.println("비겼습니다.");
} else {
System.out.println("옳은 명령어를 입력해주세요.");
}
}
}
}
public static void main(String[] args) {
Array_Repeat_Exception_Examples n = new Array_Repeat_Exception_Examples();
n.Ex16();
}
}
참고) 명품 자바 프로그래밍 3장 + 실습 문제
명품 JAVA Programming(개정4판)
명품 자바를 사랑해주시는 많은 교수님들과 독자들께 감사드립니다. 2017년 7월에 개정3판이 나오고, 두 달도 지나지 않아 Java 9가 출시되었습니다. 그리고 급기야 올해 3월에는 Java 10이 출시되었
www.booksr.co.kr